Ever wanted to build a smart system to count people entering and exiting a room or space? Meet Py-People Counter, a small yet powerful program written in Python, utilizing OpenCV for image processing. This project allows you to accurately monitor traffic flow in real-time, providing valuable data for managing crowd density and movement within a space.
In this article, we’ll walk through the Py-People Counter project, its features, how it works, and guide you on creating your own. This project is ideal for beginners interested in computer vision and people tracking or anyone looking for a solution to monitor people movement in a location.
Project Overview
Py-People Counter is a Python-based program that detects, counts, and tracks the movement of people across a defined area, counting when they enter and exit. The system uses OpenCV, a popular open-source library for computer vision, which processes video feeds from a camera and identifies people moving across the frame.
Features
- Real-time people tracking: Continuously monitors people movement in real time.
- Entry and Exit detection: Counts incoming and outgoing individuals based on their direction.
- Versatile Application: Works in various environments such as offices, malls, or event venues.
How the Py-People Counter Works
The program operates by analyzing each video frame from the camera and detecting moving objects (people) across a specific "counting line." When a person crosses this line, they are counted either as incoming or outgoing, depending on their direction.
Components of the System
- Video Capture: A camera feed provides the video input, which can be live or from a pre-recorded video file.
- Motion Detection and Tracking: OpenCV’s algorithms detect and track the motion of people across frames.
- Counting Mechanism: A line is defined in the video frame. When a person crosses it in a particular direction, they are counted as incoming or outgoing.
Setting Up the Py-People Counter
Requirements
To build and run the Py-People Counter, you’ll need:
- Python 3.x
- OpenCV: Install using
pip install opencv-python
Code Walkthrough
1. Importing Libraries
import cv2 import numpy as np
2. Defining the Counting Line
The counting line is an imaginary line drawn on the frame. We’ll monitor objects crossing this line to count them as entering or exiting.
line_position = 200 # Position of the line in the frame entry_count = 0 exit_count = 0
3. Initializing Video Capture
Load a video file or start a live feed from a connected camera.
cap = cv2.VideoCapture('path_to_video.mp4') # Replace with '0' for live camera
4. Setting Up Background Subtractor
A background subtractor helps to identify moving objects by isolating them from the static background.
bg_subtractor = cv2.createBackgroundSubtractorMOG2()
5. Processing Video Frames
For each frame, we apply the background subtractor to detect moving objects and draw bounding boxes around detected contours.
while cap.isOpened(): ret, frame = cap.read() if not ret: break # Apply background subtractor mask = bg_subtractor.apply(frame) _, mask = cv2.threshold(mask, 254, 255, cv2.THRESH_BINARY) # Find contours of detected objects contours, _ = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) for contour in contours: if cv2.contourArea(contour) > 500: # Filter small contours x, y, w, h = cv2.boundingRect(contour) cx, cy = x + w // 2, y + h // 2 # Draw bounding box cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2) # Check if person crossed the counting line if cy < line_position + 5 and cy > line_position - 5: if cx > line_position: exit_count += 1 else: entry_count += 1 # Draw counting line cv2.line(frame, (0, line_position), (frame.shape[1], line_position), (255, 0, 0), 2) # Display counts on the frame cv2.putText(frame, f"Entries: {entry_count}", (10, 50), cv2.FONT_HERSHEY_SIMPLEX, 1, (255, 255, 255), 2) cv2.putText(frame, f"Exits: {exit_count}", (10, 100), cv2.FONT_HERSHEY_SIMPLEX, 1, (255, 255, 255), 2) # Show frame cv2.imshow("People Counter", frame) if cv2.waitKey(1) & 0xFF == ord('q'): break cap.release() cv2.destroyAllWindows()
6. Running the Program
Run this script to begin counting. As people move across the frame and cross the line, their movements are detected and counted, with totals displayed on the screen.
Optimizing the Py-People Counter
This basic Py-People Counter works well in straightforward environments, but here are some ways to enhance it:
- Object Detection Algorithms: Use more advanced object detection methods, like YOLO or MobileNet, to improve accuracy.
- Fine-Tune Parameters: Adjust
contourArea
threshold, frame dimensions, and sensitivity for different environments. - Direction Detection: Use centroid tracking to track objects across multiple frames, improving direction accuracy.
Sample Use Cases
- Event Spaces: Keep track of how many people have entered and left event areas to monitor attendance and capacity.
- Retail Stores: Analyze foot traffic to improve store layout and customer experience.
- Public Transportation: Count people boarding and exiting for better crowd control and safety measures.
Conclusion
The Py-People Counter is a valuable, simple tool for counting people with minimal hardware and an easy setup. Whether it’s for events, public spaces, or personal projects, the Py-People Counter provides useful insights with only a camera and a bit of Python code. By combining computer vision with Python and OpenCV, this project offers an affordable and effective way to monitor movement and count people in real time.
For source code and updates, check out the project repository:
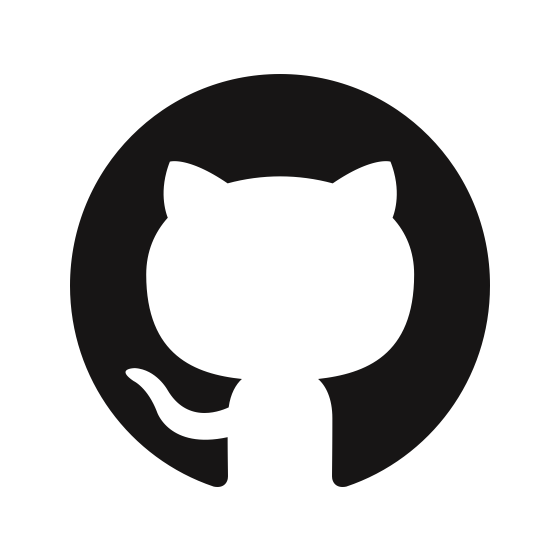