Building an Image2LCD Converter for Nokia 3310/5110 LCD Displays with Python
Introduction
The Nokia 3310/5110 LCD displays have become popular among electronics enthusiasts due to their low cost and ease of integration with microcontrollers. However, their monochrome, pixel-based display format makes it challenging to convert images directly for display.
Image2LCDConverter – a simple Python tool to convert any image into a pixel format compatible with Nokia 3310/5110 LCDs.
This article introduces the Image2LCDConverter, a Python-based project that transforms images into data arrays suitable for display on the Nokia 3310/5110 LCD. We’ll go over its features, installation, and usage, making it easy to display custom images on your retro LCD screen.
Project Overview
Image2LCDConverter is a Python script that simplifies converting images into the 84x48 pixel format used by Nokia 3310/5110 LCDs. The converter processes any input image, resizes it, converts it to monochrome, and outputs an array of binary data representing pixels, which can then be displayed on the LCD.
Key Features
- Automatic Resizing: Scales any input image to the required 84x48 pixel resolution.
- Monochrome Conversion: Converts images to black-and-white format to match the LCD’s monochrome display.
- Binary Data Output: Outputs a byte array compatible with the Nokia 3310/5110 LCD screen.
- Easy to Use: Simple interface with Python, making it easy to integrate with microcontroller projects.
Why Use Image2LCDConverter?
Displaying custom images on the Nokia 3310/5110 LCD involves transforming an image into a binary data array that the LCD can render as pixels. The Image2LCDConverter streamlines this process, allowing you to focus on building your project rather than on complex image formatting.
This tool benefits anyone interested in adding a personalized touch to projects involving the Nokia 3310/5110 LCD, including:
- Hobbyists wanting to display icons, logos, or patterns.
- Educators who want to demonstrate LCD image rendering in microcontroller courses.
- Developers working on retro-style displays and UIs.
Setting Up the Image2LCDConverter
Requirements
To get started, you’ll need:
Python 3.x: Ensure you have Python installed on your system.Pillow Library: A popular library for image processing in Python. Install it with the following command:
pip install pillow
Project Structure
The converter script includes the following key components:
- Image Loader: Loads and resizes the input image to 84x48 pixels.
- Monochrome Conversion: Converts the resized image to black and white.
- Binary Data Output: Generates binary data that matches the Nokia LCD format.
How Image2LCDConverter Works
Let’s walk through the main parts of the script, explaining how each section works to convert images for the LCD.
1. Loading and Resizing the Image
The Nokia 3310/5110 LCD resolution is fixed at 84x48 pixels, so the first step is resizing the input image to match. The Pillow
library handles this with ease:
from PIL import Image def resize_image(input_path): img = Image.open(input_path) img = img.resize((84, 48), Image.ANTIALIAS) # Resize image to LCD resolution return img
This code loads an image from input_path
and resizes it to fit the LCD screen, ensuring it’s ready for the next steps.
2. Converting the Image to Monochrome
Since the Nokia 3310/5110 LCD is monochrome, we need to convert the resized image to black and white. This process uses a threshold to determine which pixels are black (on) and which are white (off):
def convert_to_monochrome(img): return img.convert('1') # Convert image to 1-bit black and white
The convert('1')
function in Pillow
converts the image to 1-bit black-and-white, producing a binary image where each pixel is either on or off.
3. Generating the LCD-Compatible Binary Data Array
After converting the image, we generate an array of bytes that represents the pixels. Each byte contains 8 vertical pixels, which the Nokia 3310/5110 LCD interprets to fill one vertical column.
def generate_byte_array(img): byte_array = [] pixels = img.load() # Iterate over the image in blocks of 8 vertical pixels for x in range(img.width): for y in range(0, img.height, 8): byte = 0 for bit in range(8): if y + bit < img.height: # Ensure we're within bounds byte |= (1 << bit) if pixels[x, y + bit] == 0 else 0 byte_array.append(byte) return byte_array
In this function:
- We load the monochrome image and iterate through it in 8-pixel vertical slices.
- For each slice, we calculate a byte where each bit corresponds to a pixel's on/off state.
- The resulting byte array is compatible with the Nokia 3310/5110 LCD.
4. Saving the Output
Finally, we save the generated byte array to a file, which can then be used in microcontroller code to display the image on the LCD.
def save_byte_array(byte_array, output_path): with open(output_path, 'w') as f: f.write(', '.join(['0x{:02X}'.format(b) for b in byte_array]))
Putting It All Together
Here’s how the full script might look
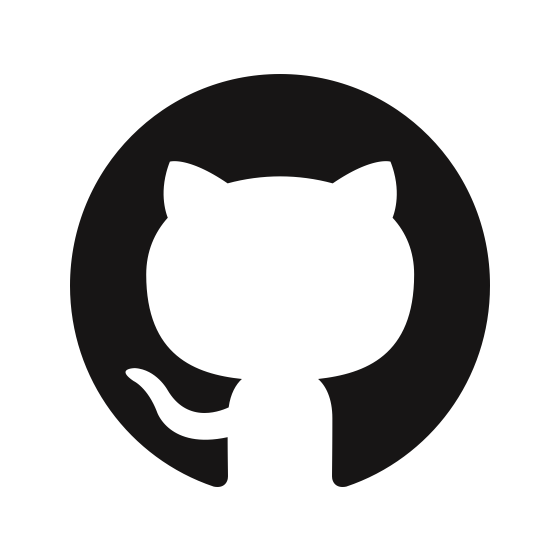
Using the Output with an Arduino
Once the byte array is generated, you can incorporate it into an Arduino sketch. Uploading the data to the microcontroller allows it to display the image on the Nokia 3310/5110 LCD.
Conclusion
The Image2LCDConverter offers a straightforward way to transform images for display on a Nokia 3310/5110 LCD. With just a few lines of Python, you can convert any image into a format that is compatible with these iconic LCDs, adding custom graphics to your embedded projects.
This project demonstrates the power of Python for image processing and embedded systems, helping makers bring retro display projects to life. If you’re ready to customize your LCDs, give the Image2LCDConverter a try!