Introduction
As solar energy continues to grow in popularity, maximizing the efficiency of solar panels is crucial for getting the most out of solar technology. Solar panels generate the most energy when they directly face the sun. However, the sun’s position changes throughout the day and across seasons. This guide will walk you through a project to build a dual-axis solar tracker using Arduino, sensors, and a motor system to keep a solar panel aligned with the brightest source of light—the sun.
Project Overview: Dual-Axis Solar Tracker
A solar tracker is a system that automatically adjusts the angle of a solar panel to follow the sun’s movement across the sky. This Arduino-based dual-axis tracker moves on both horizontal (azimuth) and vertical (elevation) axes. It uses sensors to detect the sun’s direction and adjusts the solar panel’s orientation to face the brightest light source.
Core Components:
- Arduino: The microcontroller that processes sensor data and controls the motors.
- LDR Sensors: Light-dependent resistors (LDRs) are used to detect the brightest light source.
- Servos or Stepper Motors: These motors adjust the panel's position on two axes.
- Solar Panel: The tracker is attached to a solar panel, aligning it with the sun for maximum efficiency.
- Frame: A stable frame that supports the solar panel and allows rotation along both axes.
How the Solar Tracker Works
The dual-axis solar tracker relies on four light sensors placed on the panel’s corners. These sensors detect the intensity of light at each corner, and the Arduino microcontroller compares the readings. Based on this data, the Arduino sends signals to the motors, adjusting the panel to align it precisely with the sun.
Components and Requirements
- Arduino Uno or Similar Microcontroller
- 4 Light-Dependent Resistors (LDRs): These sensors detect the intensity of light.
- 4 Resistors (e.g., 10kΩ) to connect the LDRs in a voltage divider configuration.
- 2 Servo Motors: These control movement on the horizontal and vertical axes.
- Solar Panel (small-sized for the prototype)
- Breadboard and Jumper Wires for connections
- Power Source for Arduino and motors
- Structural Frame: Material to hold the solar panel and components securely
Step-by-Step Build Guide
Step 1: Setting Up the Light Sensors
The LDRs are connected to the Arduino to measure light intensity. By placing four LDRs at the four corners of the solar panel, we can determine the direction of the sun based on the brightest detected light.
- Connect each LDR to an analog input on the Arduino through a 10kΩ resistor in a voltage divider setup.
- Connect the sensors to Arduino pins A0, A1, A2, and A3 to read their values.
Step 2: Mounting the Servo Motors
The servo motors will handle the movement on the two axes:
- Attach one motor to the base, allowing for horizontal rotation.
- Attach the other motor vertically, controlling the tilt angle of the solar panel.
Ensure the frame is stable and that the solar panel can rotate without obstruction.
Step 3: Arduino Code for Light Tracking
The Arduino code will read the LDR values and calculate adjustments for the servos. Here’s an outline of the code:
- Read Sensor Values: Continuously read the light intensity from each LDR.
- Determine Movement: Compare the LDR readings. If one side detects higher light intensity, adjust the servos to move in that direction.
- Update Motor Positions: Based on the detected differences, adjust the servos to reposition the panel.
Complete source code, wiring connection and some references can be found here in
View on GitHub
const int TOL = 5; // steps tolerance const int VERT_PADDING = 10; // vertical padding (40 in indoor testing) const int HORZ_PADDING = 20; // horizontal padding (40 in indoor testing) const int VERT_MAX_DEGREE = 120; // -160+ vertical maximum degree const int HORZ_MAX_DEGREE = 200; // -160+ horizontal maximum degree int horzDegree = 0; int vertDegree = 0; int vertPadding = 0; int horzPadding = 0; int sensorTL = A0; //LDR top left int sensorTR = A1; //LDR top right int sensorDL = A2; //LDR down left int sensorDR = A3; //LDR down right boolean RESET_DEGREE = true; //state of reset degree value if exceed maximum // motor controller pinouts //int ENA = 5; int IN1 = 6; int IN2 = 7; int IN3 = 8; int IN4 = 9; //int ENB = 10; void setup() { // initialize the serial port: Serial.begin(9600); pinMode(IN1, OUTPUT); pinMode(IN2, OUTPUT); pinMode(IN3, OUTPUT); pinMode(IN4, OUTPUT); //pinMode(ENA, OUTPUT); //pinMode(ENB, OUTPUT); initialize(); } void initialize() { // @TODO for motor calibration } void loop() { int lt = analogRead(sensorTL); // top left int rt = analogRead(sensorTR); // top right int ld = analogRead(sensorDL); // down left int rd = analogRead(sensorDR); // down right int avt = (lt + rt) / 2; // average value top int avd = (ld + rd) / 2; // average value down int avl = (lt + ld) / 2; // average value left int avr = (rt + rd) / 2; // average value right int dvert = avt - avd; // check the difference of up and down int dhoriz = avl - avr;// check the difference of left and right // -0 > dvert > 0 if (-1 * TOL > dvert || dvert > TOL) { if (avt > avd) { setVerticalSteps(true, avt - avd); } else if (avt < avd) { setVerticalSteps(false, avd - avt); } else { stopMotor(true); } } // -0 > dhorz > 0 if (-1 * TOL > dhoriz || dhoriz > TOL) { if (avl > avr) { setHorizontalSteps(false, avl - avr); } else if (avl < avr) { setHorizontalSteps(true, avr - avl); } else if (avl = avr) { stopMotor(false); } } printValues( lt, rt, ld, rd, avt, avd, avl, avr); } void setVerticalSteps(boolean up, int padding) { vertPadding = padding; if (up) { vertDegree++; if (vertDegree > VERT_MAX_DEGREE) { vertDegree = VERT_MAX_DEGREE; } } else { vertDegree--; if (vertDegree < 0) { vertDegree = 0; } } if (padding <= VERT_PADDING) { stopMotor(true); //delay(1000); } else { digitalWrite(IN1, up); digitalWrite(IN2, !up); delay(14); } } void setHorizontalSteps(boolean right, int padding) { horzPadding = padding; if (right) { horzDegree++; if (horzDegree > HORZ_MAX_DEGREE) { horzDegree = HORZ_MAX_DEGREE; } } else { horzDegree--; if (horzDegree < 0) { horzDegree = 0; } } /* if(horzDegree > HORZ_MAX_DEGREE ){ right = !right; horzDegree= horzDegree * -1; }else if(horzDegree < HORZ_MAX_DEGREE*-1){ right = !right; horzDegree= horzDegree * 1; }*/ if (padding <= HORZ_PADDING) { stopMotor(false); } else { digitalWrite(IN4, right); digitalWrite(IN3, !right); delay(14); } } void stopMotor(boolean vertical) { if (vertical == true) { digitalWrite(IN1, LOW); digitalWrite(IN2, LOW); } else { digitalWrite(IN4, LOW); digitalWrite(IN3, LOW); } if (RESET_DEGREE) { resetDegree(vertical); } } void resetDegree(boolean vertical) { if (vertical == true) { vertDegree = 0; } else { horzDegree = 0; } } void printValues(int lt, int rt, int ld, int rd, int avt, int avd, int avl, int avr) { Serial.print(vertDegree); Serial.print("\t"); Serial.print(horzDegree); Serial.print("\t"); Serial.print("\t"); Serial.print(lt); Serial.print("\t"); Serial.print(rt); Serial.print("\t"); Serial.print(ld); Serial.print("\t"); Serial.print(rd); Serial.print("\t"); Serial.print("\t"); Serial.print(avt); Serial.print("\t"); Serial.print(avd); Serial.print("\t"); Serial.print(avl); Serial.print("\t"); Serial.print(avr); Serial.print("\t"); Serial.print("\t"); Serial.print(vertPadding); Serial.print("\t"); Serial.print(horzPadding); Serial.print("\t"); Serial.println(); }
- Initial Test: Upload the code to your Arduino and place the solar tracker under a light source. The servos should adjust the panel to face the light.
- Calibration: Adjust the light sensor thresholds in the code if the movement is too sensitive or slow.
- Outdoor Testing: Place the tracker in sunlight to see how well it follows the sun.
Advantages of Dual-Axis Tracking
- Increased Energy Capture: Tracking the sun on two axes allows for continuous adjustment, maximizing the panel's exposure.
- Higher Efficiency: Dual-axis trackers can significantly increase solar panel efficiency, capturing up to 40% more energy than stationary panels.
Conclusion
Building a dual-axis solar tracker with Arduino is a rewarding project that demonstrates how technology can enhance the efficiency of renewable energy sources. This system can be scaled for larger solar panels, providing an affordable, energy-efficient solution to capture the sun’s power more effectively. Whether for personal use or as a classroom project, this solar tracker offers a practical approach to learning about both electronics and solar energy.
Source Code: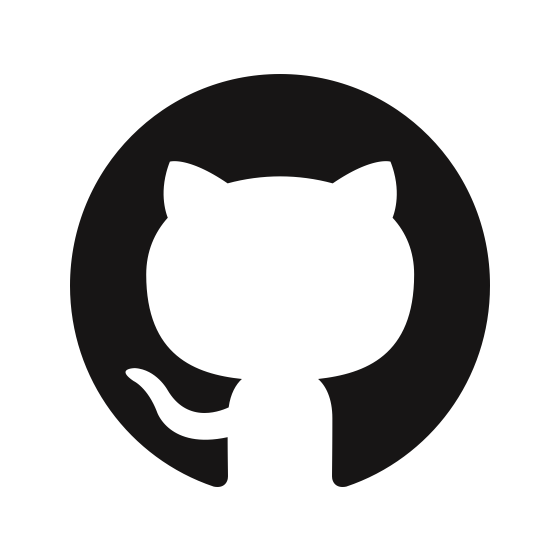